Results for
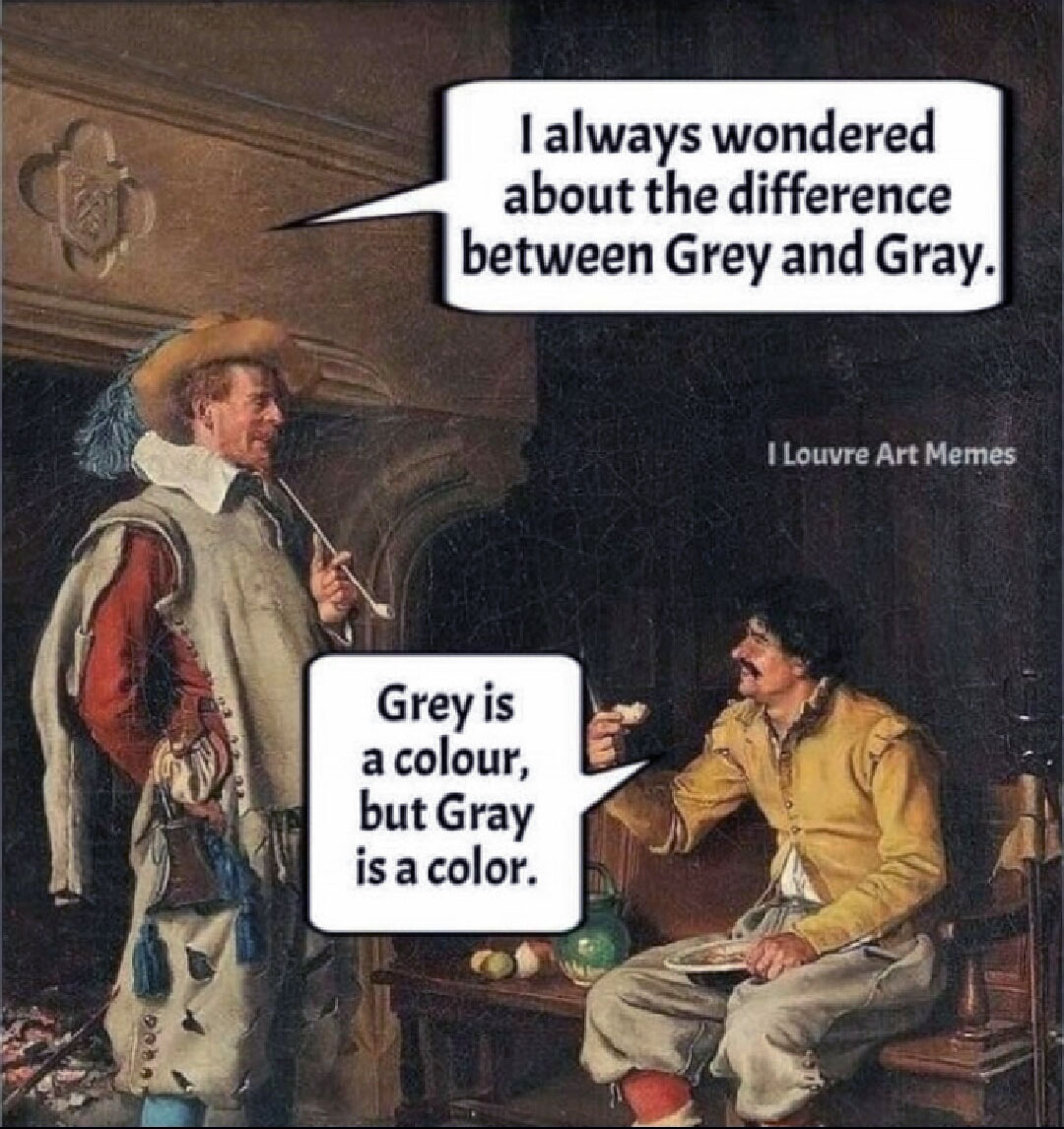
The Queen’s MATLAB
Good news for those of you who speak (and spell) the Queen's English instead of the US dialect. We are working night and day to make MATLAB use equally satisfying for the entire world's English speaking population!ContentsExpectations for Upcoming ReleasesNamesOur Current ListHelp Us Out!Expectations for Upcoming ReleasesYou can expect, in upcoming releases, to create a colorbar or a colourbar.
- Channel sounding (for accurate distance measurements)
- Decision-based advertising filtering (for more efficient channel scanning)
- Monitoring advertisers (for improved energy efficiency when devices come into and go out of range)
- Frame space updates (for both higher throughput and better coexistence management)
- Keyless vehicle entry, performed by communication between a key fob or phone and the car’s anchor points
- Smart locks, to permit access only when an authorized device is within a designated proximity to the locks
- Geofencing, to limit access to designated areas
- Warehouse management, to monitor inventory and manage logistics
- Asset tracking for virtually any object of interest

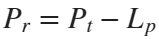
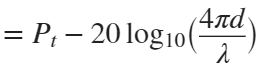








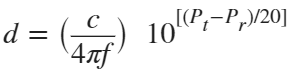
- Round-trip time (RTT) measurement
- Phase-based ranging (PBR) measurement

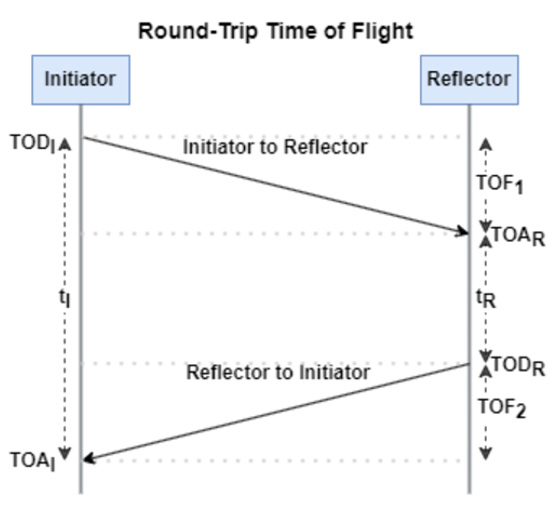










- High Data Throughput, up to 8 Mbps
- 5 and 6 GHz operation
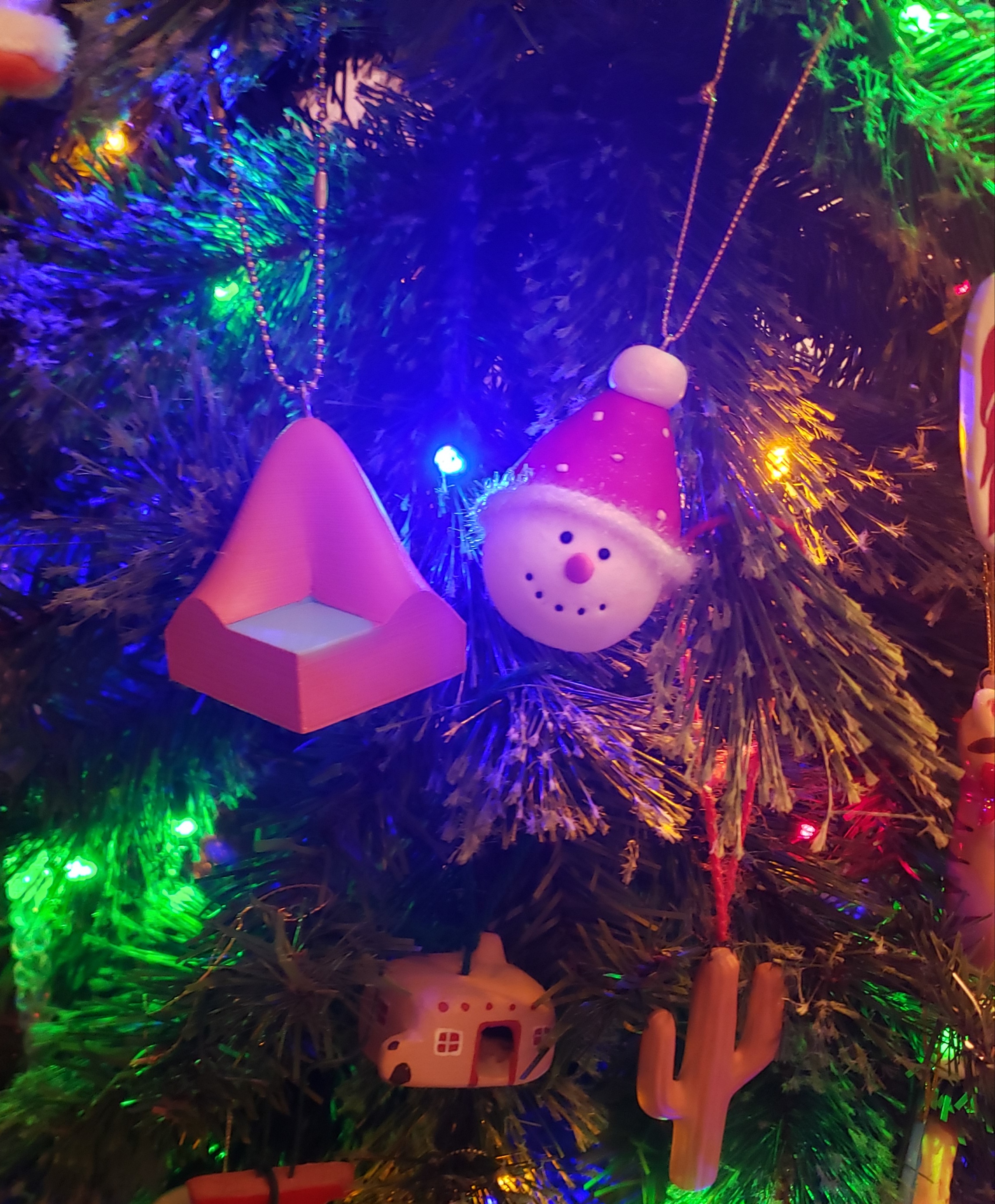

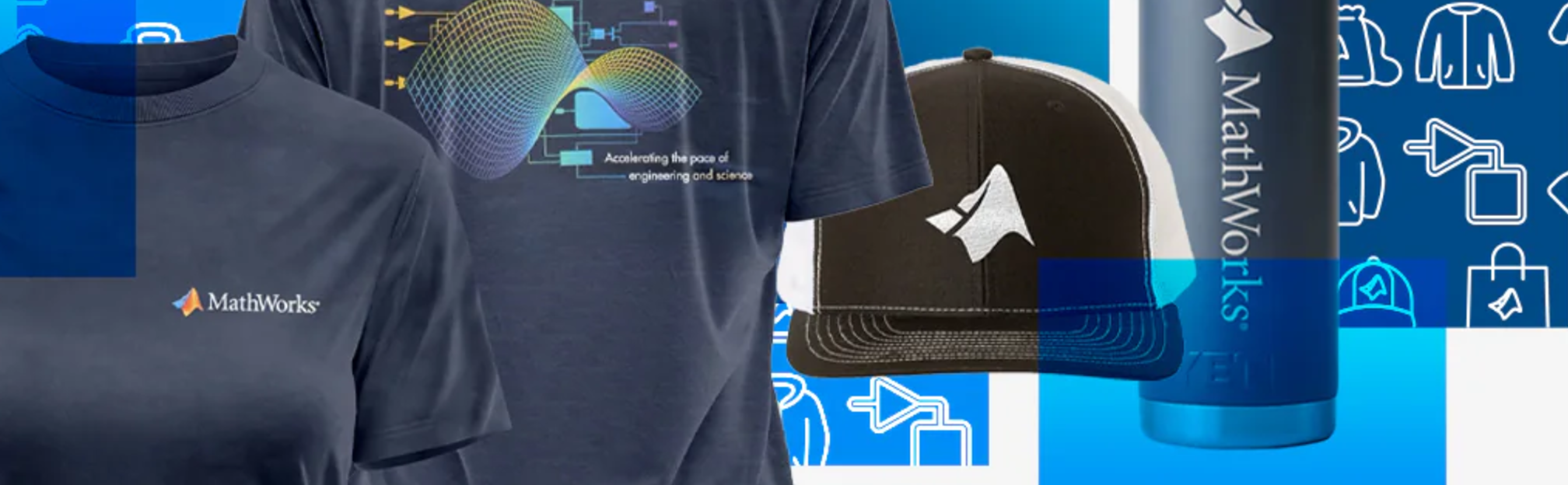
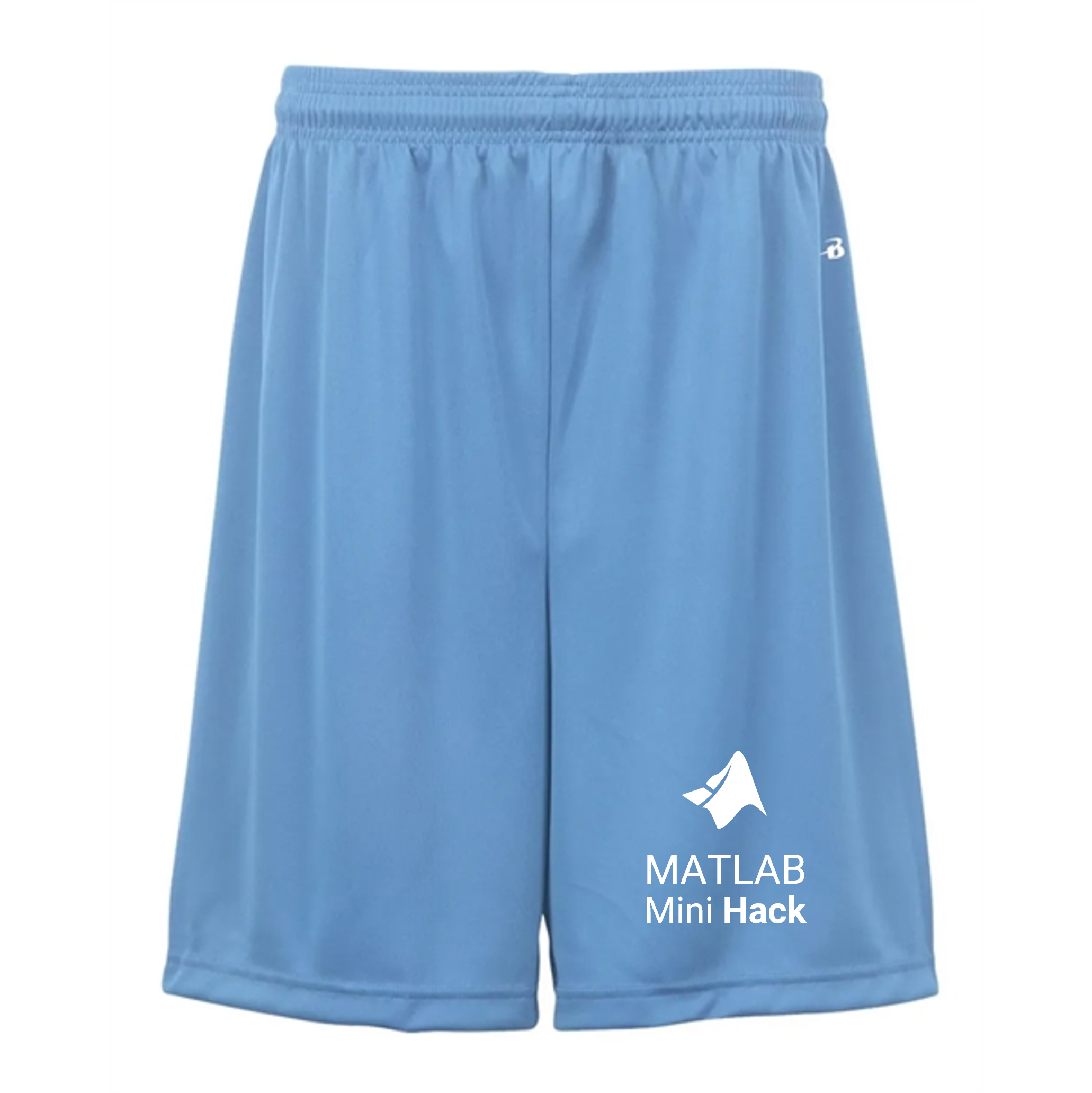
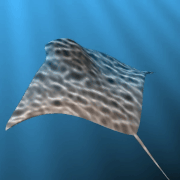
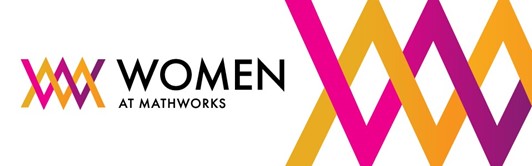
- fstr: a Python f-string like expression
- printf: an easy to use fprintf function, accepts multiple arguments with seperator, end string control.
- clc - clears command window, workspace not affected
- clear - clears all variables from workspace, all variable values are lost
- diary - records into a file almost everything that appears in command window.
- exit - exits the MATLAB session
- who - prints all variables in the workspace
- whos - prints all variables in current workspace, with additional information.
- Mathematical constants: pi, i, j, Inf, Nan, realmin, realmax
- Precedence rules: (), ^, negation, */, +-, left-to-right
- and, or, not, xor
- exp - exponential
- log - natural logarithm
- log10 - common logarithm (base 10)
- sqrt (square root)
- fprintf("final amount is %f units.", amt);
- can have: %f, %d, %i, %c, %s
- %f - fixed-point notation
- %e - scientific notation with lowercase e
- disp - outputs to a command window
- % - fieldWith.precision convChar
- MyArray = [startValue : IncrementingValue : terminatingValue]
- Transpose an array with []'
- isinf(A) - check if the array elements are infinity
- isnan(A)
- ceil(x) - rounds each element of x to nearest integer >= to element
- floor(x) - rounds each element of x to nearest integer <= to element
- fix(x) - rounds each element of x to nearest integer towards 0
- round(x) - rounds each element of x to nearest integer. if round(x, N), rounds N digits to the right of the decimal point.
- rem(dividend, divisor) - produces a result that is either 0 or has the same sign as the dividen.
- mod(dividend, divisor) - produces a result that is either 0 or same result as divisor.
- Ex: 12/2, 12 is dividend, 2 is divisor
- sum(inputArray) - sums all entires in array
- abs(z) - absolute value, is magnitude of complex number (phasor form r*exp(j0)
- angle(z) - phase angle, corresponds to 0 in r*exp(j0)
- complex(a,b) - creates complex number z = a + jb
- conj(z) - given complex conjugate a - jb
- real(z) - extracts real part from z
- imag(z) - extracts imaginary part from z
- unwrap(z) - removes the modulus 2pi from an array of phase angles.
- mean(xAr) - arithmetic mean calculated.
- std(xAr) - calculated standard deviation
- median(xAr) - calculated the median of a list of numbers
- mode(xAr) - calculates the mode, value that appears most often
- max(xAr)
- min(xAr)
- If using &&, this means that if first false, don't bother evaluating second
- rand(numRand, 1) - creates column array
- rand(1, numRand) - creates row array, both with numRand elements, between 0 and 1
- randi(maxRandVal, numRan, 1) - creates a column array, with numRand elements, between 1 and maxRandValue.
- randn(numRand, 1) - creates a column array with normally distributed values.
- Ex: 10 * rand(1, 3) + 6
- "10*rand(1, 3)" produces a row array with 3 random numbers between 0 and 10. Adding 6 to each element results in random values between 6 and 16.
- randi(20, 1, 5)
- Generates 5 (last argument) random integers between 1 (second argument) and 20 (first argument). The arguments 1 and 5 specify a 1 × 5 row array is returned.
- primes(maxVal) - returns prime numbers less than or equal to maxVal
- isprime(inputNums) - returns a logical array, indicating whether each element is a prime number
- factor(intVal) - returns the prime factors of a number
- gcd(aVals, bVals) - largest integer that divides both a and b without a remainder
- lcm(aVals, bVals) - smallest positive integer that is divisible by both a and b
- factorial(intVals) - returns the factorial
- perms(intVals) - returns all the permutations of the elements int he array intVals in a 2D array pMat.
- randperm(maxVal)
- nchoosek(n, k)
- binopdf(x, n, p)
- cat, vertcat, horzcat
- Flattening an array, becomes vertical: sampleList = sampleArray ( : )
- nLargest = length(inArray) - number of elements along largest dimension
- nDim = ndims(inArray)
- nArrElement = numel(inArray) - nuber of array elements
- [nRow, nCol] = size(inArray) - returns the number of rows and columns on array. use (inArray, 1) if only row, (inArray, 2) if only column needed
- aZero = zeros(m, n) - creates an m by n array with all elements 0
- aOnes = ones(m, n) - creates an m by n array with all elements set to 1
- aEye = eye(m, n) - creates an m by n array with main diagonal ones
- aDiag = diag(vector) - returns square array, with diagonal the same, 0s elsewhere.
- outFlipLR = fliplr(A) - Flips array left to right.
- outFlipUD = flipud(A) - Flips array upside down.
- outRot90 = rot90(A) - Rotates array by 90 degrees counter clockwise around element at index (1,1).
- outTril = tril(A) - Returns the lower triangular part of an array.
- outTriU = triu(A) - Returns the upper triangular part of an array.
- arrayOut = repmat(subarrayIn, mRow, nCol), creates a large array by replicating a smaller array, with mRow x nCol tiling of copies of subarrayIn
- reshapeOut - reshape(arrayIn, numRow, numCol) - returns array with modifid dimensions. Product must equal to arrayIn of numRow and numCol.
- outLin = find(inputAr) - locates all nonzero elements of inputAr and returns linear indices of these elements in outLin.
- [sortOut, sortIndices] = sort(inArray) - sorts elements in ascending order, results result in sortOut. specify 'descend' if you want descending order. sortIndices hold the sorted indices of the array elements, which are row indices of the elements of sortOut in the original array
- [sortOut, sortIndices] = sortrows(inArray, colRef) - sorts array based on values in column colRef while keeping the rows together. Bases on first column by default.
- isequal(inArray1, inarray2, ..., inArrayN)
- isequaln(inArray1, inarray2, ..., inarrayn)
- arrayChar = ischar(inArray) - ischar tests if the input is a character array.
- arrayLogical = islogical(inArray) - islogical tests for logical array.
- arrayNumeric = isnumeric(inArray) - isnumeric tests for numeric array.
- arrayInteger = isinteger(inArray) - isinteger tests whether the input is integer type (Ex: uint8, uint16, ...)
- arrayFloat = isfloat(inArray) - isfloat tests for floating-point array.
- arrayReal= isreal(inArray) - isreal tests for real array.
- objIsa = isa(obj,ClassName) - isa determines whether input obj is an object of specified class ClassName.
- arrayScalar = isscalar(inArray) - isscalar tests for scalar type.
- arrayVector = isvector(inArray) - isvector tests for a vector (a 1D row or column array).
- arrayColumn = iscolumn(inArray) - iscolumn tests for column 1D arrays.
- arrayMatrix = ismatrix(inArray) - ismatrix returns true for a scalar or array up to 2D, but false for an array of more than 2 dimensions.
- arrayEmpty = isempty(inArray) - isempty tests whether inArray is empty.
- primeArray = isprime(inArray) - isprime returns a logical array primeArray, of the same size as inArray. The value at primeArray(index) is true when inArray(index) is a prime number. Otherwise, the values are false.
- finiteArray = isfinite(inArray) - isfinite returns a logical array finiteArray, of the same size as inArray. The value at finiteArray(index) is true when inArray(index) is finite. Otherwise, the values are false.
- infiniteArray = isinf(inArray) - isinf returns a logical array infiniteArray, of the same size as inArray. The value at infiniteArray(index) is true when inArray(index) is infinite. Otherwise, the values are false.
- nanArray = isnan(inArray) - isnan returns a logical array nanArray, of the same size as inArray. The value at nanArray(index) is true when inArray(index) is NaN. Otherwise, the values are false.
- allNonzero = all(inArray) - all identifies whether all array elements are non-zero (true). Instead of testing elements along the columns, all(inArray, 2) tests along the rows. all(inArray,1) is equivalent to all(inArray).
- anyNonzero = any(inArray) - any identifies whether any array elements are non-zero (true), and false otherwise. Instead of testing elements along the columns, any(inArray, 2) tests along the rows. any(inArray,1) is equivalent to any(inArray).
- logicArray = ismember(inArraySet,areElementsMember) - ismember returns a logical array logicArray the same size as inArraySet. The values at logicArray(i) are true where the elements of the first array inArraySet are found in the second array areElementsMember. Otherwise, the values are false. Similar values found by ismember can be extracted with inArraySet(logicArray).
- any(x) - Returns true if x is nonzero; otherwise, returns false.
- isnan(x) - Returns true if x is NaN (Not-a-Number); otherwise, returns false.
- isfinite(x) - Returns true if x is finite; otherwise, returns false. Ex: isfinite(Inf) is false, and isfinite(10) is true.
- isinf(x) - Returns true if x is +Inf or -Inf; otherwise, returns false.
- fctName = @(arglist) expression - anonymous function
- nargin - keyword returns the number of input arguments passed to the function.
- continue: Skips the rest of the current loop iteration and begins the next iteration.
- break: Exits a loop before it has finished all iterations.
- You are allowed to have nested functions in MATLAB
- fctName = @(argList) expression
- Ex: RaisedCos = @(angle) (cosd(angle))^2;
- global variables - can be accessed from anywhere in the file
- Persistent variables
- persistent variable - only known to function where it was declared, maintains value between calls to function.
- Recursion - base case, decreasing case, ending case
- nargin - evaluates to the number of arguments the function was called with
- plot(xArray, yArray)
- refer to help plot for more extensive documentation, chapter 12 only briefly covers plotting
- doing strings(sz) returns an array of strings with no characters, where sz defines the size.
- strExp = sprintf("%0.6e", pi)